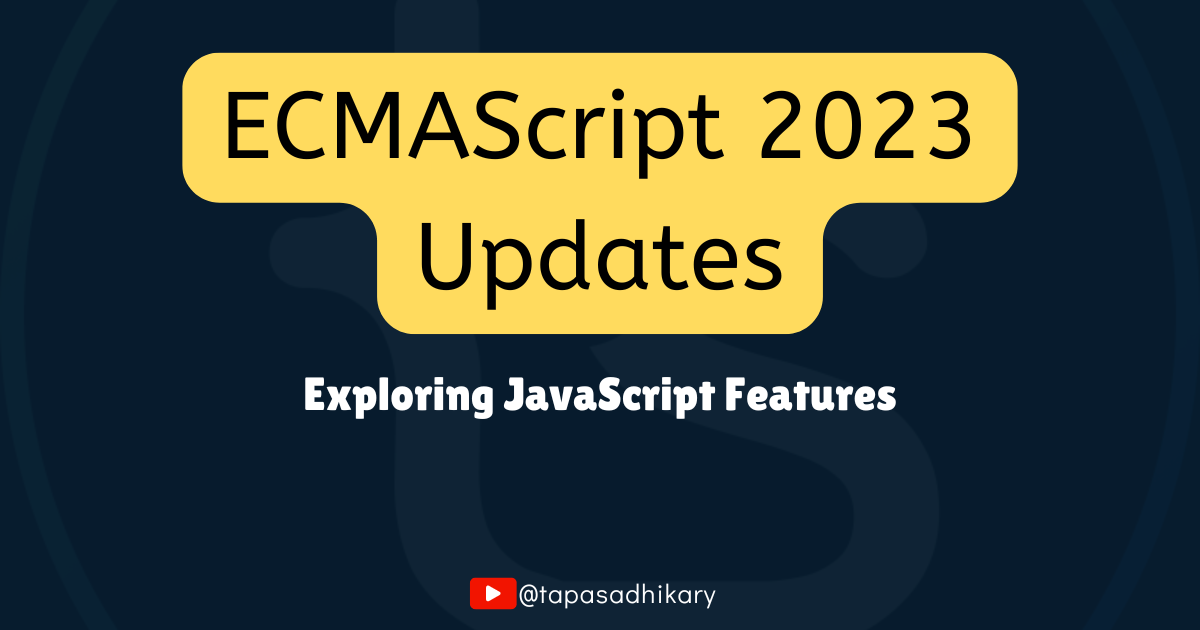
Exploring Advanced JavaScript Features: A Deep Dive into ECMAScript 2023 Updates
Learn about the addition of latest features in ECMAScript 2023.
ECMAScript, also known as the backbone of JavaScript, has come a long way since its birth at Netscape in 1995. Within two years, it was standardized as ECMAScript 1.
It only took ECMAScript 4 years to reach its third generation. By 1999, it had gained new features like regular expressions and better error handling.
Following the rapid growth, a major update was proposed in the early 2000s. Unfortunately, it was delayed due to conflicts and disagreements among the developers. However, things picked up again in 2009 with ES5. ECMAScript 5 brought strict mode, JSON support, and even better object handling.
The real game changer was ECMAScript 6 in 2015. ES6 was especially powerful because it introduced simpler ways to write functions. It added classes, modules, and all sorts of things to make it less monotonous. Since then, new versions have come out every year. Notable updates are the 2017 async/await update, easier imports (2020), and top-level await (2022). The latest 2023 update has a range of fresh features.
What's New in ECMAScript 2023?
ECMAScript 2023 brings some great new features to JavaScript. Which makes it easier to work with arrays, handle Unicode characters, and write asynchronous code. These updates help developers write code that's more readable and easier to manage.
ES14 also improved regular expressions with the new RegExp v Flag, which enables more knowledgeable pattern matching (e.g. set notation and nested character classes)
When there are secure and fast shared hosting solutions to boost the performance of a website, similar to all these updates that JS has lately been going through — they make it more web global.
Array Methods
ES14 introduces us to multiple new array methods in JavaScript. Here's a breakdown:
findLast()
Purpose: Returns the final element in an array that fulfils the criteria of a specified test function.
Example:
const scores = [90, 85, 95, 70, 80]; const lastScoreAbove80 = scores.findLast(score => score > 80); console.log(lastScoreAbove80); // Output: 95
findLastIndex()
Purpose: Finds the position of the last element in an array that meets the conditions of a given test function; if no elements match, it returns
-1
.Example:
const scores = [90, 85, 95, 70, 80]; const lastIndexAbove80 = scores.findLastIndex(score => score > 80); console.log(lastIndexAbove80); // Output: 2 (index of element 95)
toReversed()
Purpose: Generates a new array with the elements arranged in reverse, without modifying the original array.
Example:
const letters = ["a", "b", "d", "f"]; const reversedLetters = letters.toReversed(); console.log(reversedLetters); // Output: ["f", "d", "b", "a"]
toSorted()
Purpose: Creates a new array with the elements sorted in order. The original array remains unchanged.
Example:
const numbers = [4, 2, 3, 1]; const sortedNumbers = numbers.toSorted(); console.log(sortedNumbers); // Output: [1, 2, 3, 4]
toSpliced()
Purpose: Generates a new array by adding or removing specified elements. The original array is left unmodified.
Example:
const months = ["Jan", "Feb", "Mar", "Apr"]; const splicedMonths = months.toSpliced(1, 1); // Removes "Feb" console.log(splicedMonths); // Output: ["Jan", "Mar", "Apr"]
with()
Purpose: Creates a new array with updated elements while keeping the original array unchanged. This ensures safe and non-destructive modifications.
Example:
const months = ["January", "February", "April"]; const updatedMonths = months.with(2, "Apr"); console.log(updatedMonths); // Output: ["January", "February", "Apr"]
You can learn more about the
with()
method from here.
Unicode Advancements
ECMAScript 2023 (ES14) improves how JavaScript handles Unicode with Well-Formed Unicode Strings. This feature makes sure that text across different languages is correctly formatted. To reduce errors, it adds two new methods:
String.prototype.isWellFormed
: Checks if a string is correctly formatted.String.prototype.toWellFormed
: Fixes incorrectly formatted strings by replacing invalid characters.
Shebang
The shebang syntax in ECMAScript 2023 (ES14) is the very first thing that allows JavaScript files to be executed directly as scripts in environments that support it such as Unix-like operating systems.
Structure: #!/path/to/interpreter [optional-arg]
Example: #!/usr/bin/env node
This line indicates that the script should be executed using the Node.js interpreter. When the script file is run with a command like ‘./fileName.js’, the operating system reads the shebang and invokes the specified interpreter to execute the code that follows.
Three points to note:
Placement: The shebang must be at the top of the file, with no preceding whitespace.
Execution: To run a JavaScript file with a shebang, ensure it has executable permissions (using chmod ‘+x fileName.js’), allowing you to execute it directly from the command line.
Standardization: This feature enhances portability and usability of JavaScript scripts across different environments, aligning JavaScript more closely with other scripting languages that utilize shebangs.
Compatibility Overview
ECMAScript 2023 has brought in major developments. However, just like any groundbreaking achievement, there's this compatibility concern developers are getting worked up on. Here are some aspects to mull over:
General Browser Support: Most modern browsers and JavaScript engines are working on adding ES14 features, but not all of them are fully supported yet. It’s a good idea for developers to double-check which features are compatible with the browsers they need to support since some may still be catching up.
GraalVM Compatibility: GraalVM is fully up to date with ECMAScript 2023. So it lets developers use the newest JavaScript features in a stable environment. Developers can now also choose which ECMAScript version their applications should use. This makes it easy to take advantage of new features while keeping compatibility with older code.
Framework and Library Updates: Major frameworks and libraries are steadily rolling out updates to include ES14 features. Tools like Angular and BugSnag, for example, are working to ensure they support the latest JavaScript standards. So that developers can use new features without losing performance or stability.
Adaptation Strategies
Since not all platforms support these new features right away, it's essential to adopt strategies that help your applications run smoothly everywhere. You can approach it this way:
Check for Feature Support: Use feature detection in your applications to check if certain ES14 features are supported in a given environment. If they aren’t, you can provide alternatives or polyfills. Polyfills make sure your app runs smoothly no matter where it’s used.
Use Transpilers: Tools like Babel or TypeScript can convert ES14 code into older JavaScript versions. This lets you take advantage of the latest features while still supporting older browsers that don’t recognize them.
Test on Different Platforms: Regularly test your applications across multiple browsers and environments to catch any compatibility issues early on. This way, you can fix problems before they affect users and keep the experience smooth.
Tips to Avoid Potential Pitfalls
We can suggest some advice that should help you avoid common issues when using Babel to transpile modern JavaScript features:
Integrate with Build Tools: Use bundlers like Webpack, Rollup, or Parcel to integrate Babel into your build process. Configure these tools to handle transpilation and polyfill loading efficiently during the build step.
Optimize Polyfill Usage: Use tools like core-js to load only the necessary polyfills based on your target environments. Avoid loading unnecessary polyfills by specifying only the features your application actually needs.
Configure Babel Correctly: Define your target browsers or environments in your Babel setup using tools like browserslist or
@babel/preset-env
. Make sure you enable all necessary plugins, especially if you need to support older browsers like Internet Explorer 8.Use Feature Detection: Check for feature support in the browser before using newer JavaScript features. You can conditionally apply polyfills or provide alternative code when a feature isn't available.
Test in Different Environments: Test your application regularly across multiple browsers and environments to catch compatibility issues early. Use tools like BrowserStack or Sauce Labs to ensure your app works well on various devices and browsers.